In the world of Linux administration, efficiency is everything. For those managing complex systems or juggling repetitive tasks, the struggle to save time while ensuring consistency can feel like an uphill battle. Whether it’s organising files, monitoring server performance, or scheduling backups, even the most seasoned administrators can find themselves bogged down by mundane yet critical responsibilities.
That’s where Linux shell scripting steps in. As a powerful tool that transforms sequences of commands into automated workflows, shell scripting is the ultimate ally in reclaiming time and reducing errors. It empowers administrators to execute tasks with precision, scalability, and unmatched simplicity. But where do you start? And how do you make the most of shell scripting’s capabilities without feeling overwhelmed by its technical depth?
This guide is here to answer those questions. You’ll discover practical insights, master essential scripting concepts, and explore real-world examples designed to make Linux automation accessible and impactful. Whether you’re automating file management, system monitoring, or user administration, this post will equip you with the tools and techniques to streamline your workflows and elevate your efficiency.
What Is Linux Shell Scripting?
Linux shell scripting is the process of creating a script—a series of commands written in a text file—to automate tasks in a Linux environment. These scripts use shell interpreters like Bash, Zsh, or Dash to execute commands sequentially. For instance, a simple script could clean temporary files by executing a command like rm -rf /tmp/* regularly. With more advanced scripts, you can perform tasks like data backups, user account creation, or system updates.
According to a report on linuxconfig.org, scripts are commonly used to automate performance monitoring. For example, a script monitoring CPU and memory usage ensures optimal system performance while reducing manual intervention. This showcases the immense value shell scripting adds to Linux administration.
Shell scripting is indispensable in Linux administration because it bridges the gap between manual operations and fully automated processes. By leveraging loops (for, while), conditional statements (if, case), and functions, you can create powerful, reusable scripts to manage complex systems efficiently. For example, a script can monitor system logs for errors and automatically notify administrators via email.
The beauty of shell scripting lies in its versatility. Administrators can adapt scripts to unique requirements, ensuring consistent and reliable performance. Whether it’s executing routine tasks or managing intricate workflows, shell scripting offers a way to simplify Linux administration while reducing the potential for human error.
Why Automate Tasks with Shell Scripts?
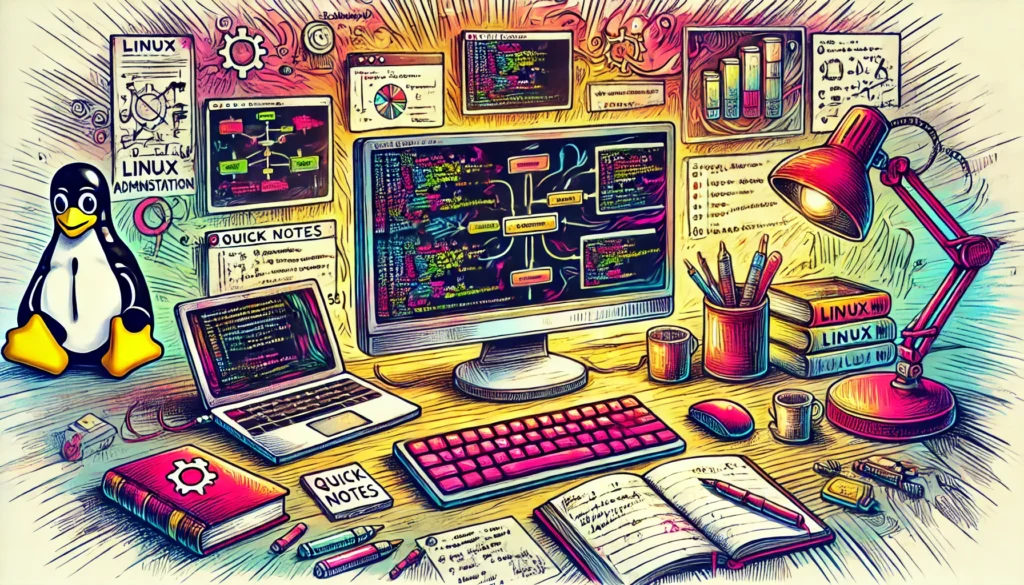
Automation in Linux administration is essential for managing repetitive and time-consuming tasks. Shell scripts allow administrators to execute these tasks with minimal manual intervention, saving time and reducing errors. For example, consider a situation where you need to back up a directory daily. Writing a script with rsync can automate this process and ensure it runs on schedule using cron.
Another key advantage of automation is consistency. Tasks like creating user accounts, applying security patches, or monitoring disk usage are prone to human error when done manually. A shell script can execute these actions uniformly every time, ensuring compliance with organisational policies.
Automation also improves scalability. In environments with multiple servers, a single script can be deployed across systems, handling tasks such as log rotation or resource monitoring efficiently. For example, a script can check CPU usage across servers and generate a consolidated report, providing administrators with actionable insights.
By automating tasks with shell scripts, administrators can focus on higher-value activities, such as system optimisation or implementing new technologies. In today’s fast-paced IT landscape, automation isn’t just a convenience; it’s a necessity for effective Linux administration.
Essential Shell Scripting Concepts
To write effective shell scripts, it’s crucial to understand some foundational concepts. These include variables, control structures, and functions, which form the backbone of any script.
1. Variables: Variables store data that can be used and modified within a script. For example:
#!/bin/bash
backup_dir="/backup"
echo "Backing up to $backup_dir"
This script assigns a directory path to a variable and references it later.
2. Control Structures: Conditional statements like if and case allow scripts to make decisions based on specific conditions. Loops, such as for and while, enable repetitive tasks. Example:
#!/bin/bash
for file in /tmp/*; do
echo "Deleting $file"
rm -f "$file"
done
This script iterates through files in /tmp and deletes them.
3. Functions: Functions allow you to group reusable code blocks. For example:
#!/bin/bash
backup() {
rsync -av /source /destination
echo "Backup completed"
}
backup
By combining these elements, you can write modular and maintainable scripts that handle complex tasks effectively. Additionally, testing and debugging scripts are essential to ensure they run as expected.
Tools and Techniques to Enhance Shell Scripting
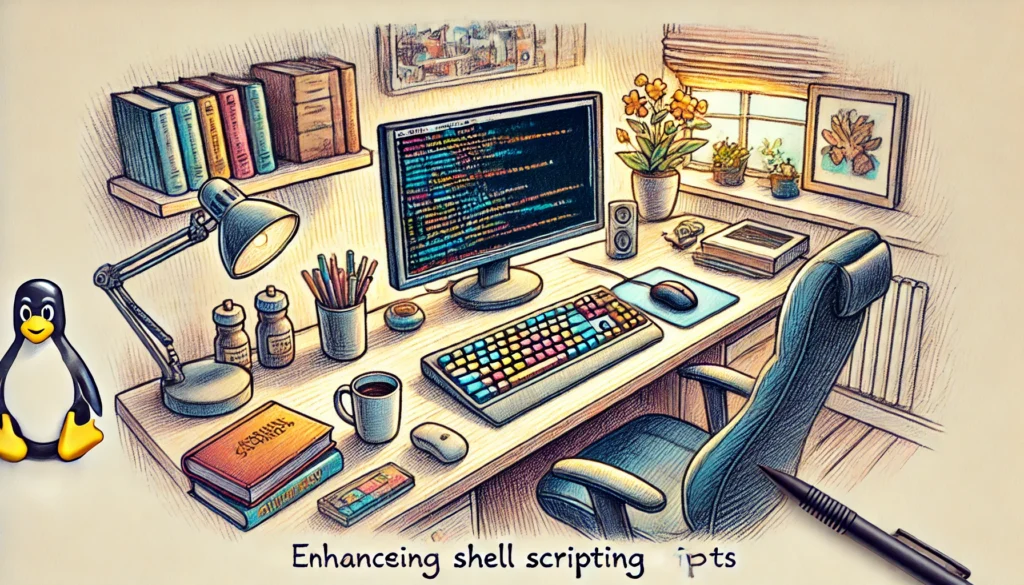
Enhancing your shell scripting capabilities involves leveraging powerful tools and employing best practices. Here are some essential tools and techniques:
1. Command-Line Utilities:
- grep: Search for patterns within files or output streams.
- sed and awk: Perform advanced text processing.
- cron: Schedule scripts to run at specific intervals.
- xargs: Execute commands in parallel, improving performance.
2. Debugging and Error Handling:
- Use set -e to terminate a script if any command fails.
- Redirect error messages to a log file for easier debugging.
- Include exit codes to indicate success or failure.
3. Advanced Techniques:
- Parallel processing: Use tools like xargs or GNU parallel for faster execution of repetitive tasks.
- Integration: Combine shell scripts with Python or other languages for more complex workflows.
- Modularisation: Break scripts into smaller, reusable components.
For instance, you can use grep and awk to extract specific log entries and process them efficiently:
grep "ERROR" /var/log/syslog | awk '{print $1, $2, $3}' > error_report.txt
By mastering these tools and techniques, you can significantly enhance the functionality and reliability of your scripts.
Practical Examples of Automated Tasks
1. Automating Backups: A script using rsync can back up a directory to a remote server:
#!/bin/bash
rsync -av /data /backup/server
Scheduling this script with cron ensures it runs daily without manual intervention.
2. Monitoring System Logs: A script can search for critical errors in logs and send an email alert:
#!/bin/bash
grep "ERROR" /var/log/syslog > /tmp/errors.log
mail -s "Critical Errors Found" admin@example.com < /tmp/errors.log
3. Disk Space Monitoring: This script checks disk usage and alerts when it exceeds 90%:
#!/bin/bash
df -h | awk '$5 > 90 {print $0}' | mail -s "Disk Usage Alert" admin@example.com
These examples demonstrate how shell scripting simplifies everyday tasks, allowing administrators to focus on critical issues.
Common Mistakes and How to Avoid Them
Even experienced administrators can make mistakes when scripting. Here are common pitfalls and ways to avoid them:
- Lack of Documentation: Without comments, scripts become difficult to understand and maintain. Always include clear comments.
- Hard-Coding Values: Avoid embedding static values in scripts. Use variables instead, making scripts more flexible.
- Ignoring Error Handling: Ensure scripts handle errors gracefully. Use conditional checks and redirect errors to log files. According to moldstud.com, error handling remains one of the most overlooked aspects of scripting.
- Insufficient Testing: Test scripts in a controlled environment before deploying them in production. Neglecting this step often results in unexpected behaviour.
- Overcomplicating Scripts: Keep scripts simple and modular. Break complex tasks into smaller functions. A report from thenucleargeeks.com highlights that excessive complexity is a frequent issue that reduces maintainability.
By addressing these issues, you’ll create robust and reliable scripts that enhance your Linux administration workflows.
The Future of Shell Scripting in Linux Administration
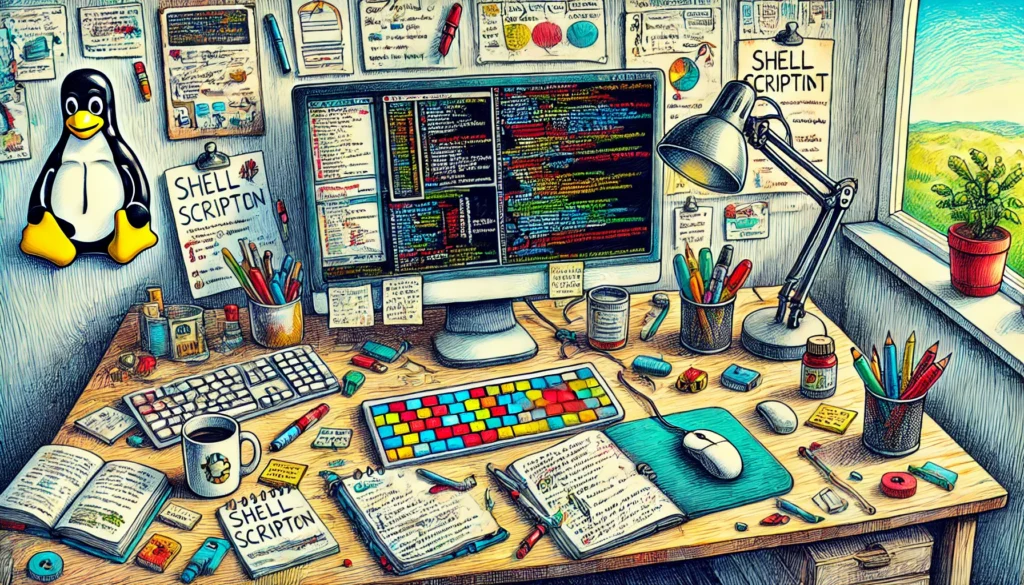
Shell scripting remains a cornerstone of Linux administration, even as new technologies emerge. Its simplicity and versatility make it ideal for automating tasks in both traditional and modern IT environments.
As DevOps practices gain traction, shell scripting integrates seamlessly into CI/CD pipelines, automating deployments, testing, and monitoring. Scripts can also interact with APIs, enabling administrators to manage cloud infrastructure efficiently.
Moreover, the rise of containerisation tools like Docker has expanded scripting’s role. Shell scripts can build, deploy, and manage containers, streamlining application development and deployment.
While higher-level programming languages offer additional capabilities, shell scripting’s lightweight nature ensures it remains an indispensable tool for quick, system-level tasks. Administrators who master shell scripting will find themselves well-equipped to navigate the evolving landscape of Linux administration.
The future of shell scripting lies in its adaptability. As IT environments grow more complex, the demand for simple, reliable automation solutions ensures shell scripting’s continued relevance and importance.
Take the First Step Towards Automation Mastery
Mastering Linux shell scripting can transform how you manage systems and solve administrative challenges. By embracing automation, you can save time, reduce errors, and unlock the potential to focus on strategic initiatives.
Begin by identifying repetitive tasks in your workflows and scripting solutions to simplify them. Start small, leveraging the examples and techniques shared here, and build your expertise step by step. For those ready to dive deeper, consider enrolling in a comprehensive Linux scripting course or accessing curated resources from trusted platforms like LinuxConfig and MoldStud.
Don’t let mundane tasks hold you back. Take control of your Linux systems, streamline your processes, and drive efficiency with the power of shell scripting. The possibilities are endless—all you need is the right approach and tools to get started.